Salesforce Canvas App is used to integrate a third-party application in Salesforce. The third-party app that you want to expose as a canvas app can be written in any language. The only requirement is that the app has a secure URL (HTTPS). This post is about Salesforce Canvas App Integration with Nodejs Application osted on localhost. You would first need to install the certificates to run the app in salesforce to avoid getting SSL error.
1. Create connected app in Salesforce. Refer blog to create a canvas connected app. Copy the consumer secret to store in node.js app for authentication
2. Create a node.js app. Refer blog on how to create node.js app and host in localhost
- Create a genSSL.sh file and write the below shell script to generate the certificates
openssl genrsa -out ssl-key.pem 1024
openssl req -new -key ssl-key.pem -out certrequest.csr
openssl x509 -req -in certrequest.csr -signkey ssl-key.pem -out ssl-cert.pem -days 365
cp ssl-cert.pem ssl-cert.cer
- Run the shell script to generate certificates and install the certificates. After installation the project structure will be as below

- Create a config.js file in your folder and store the consumer secret received from salesforce
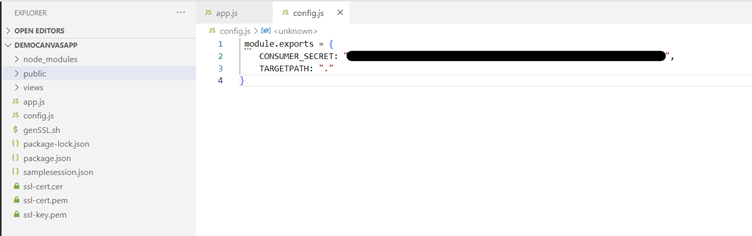
- Download the Canvas SDK from force.com github and place it in public folder
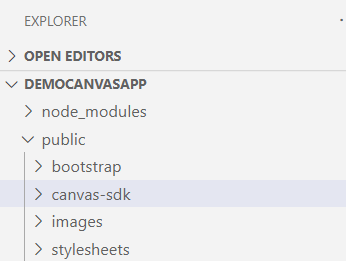
- Install the express session and body parser using terminal
- npm install express-session
- npm install body-parser
- In app.js file add app.post() method to authenticate the signed request send from salesforce
- Run node app.js in terminal
- Navigate to Salesforce and open your canvas app
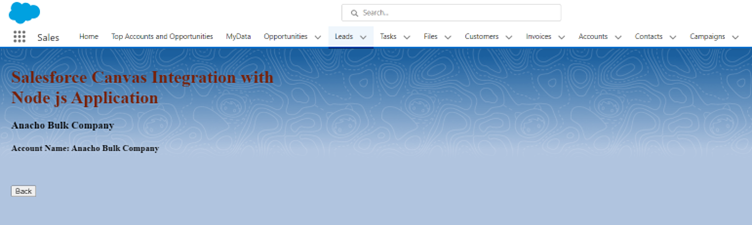
- Below is the code for app.js
// Requiring module
const crypto = require("crypto");
const express = require('express');
const https = require('https');
const fs = require('fs');
const path = require('path');
const config = require("./config.js");
let envelope;
// Port Number
const PORT = process.env.PORT ||4884;
// Creating express object
const app = express();
// set all environments
app.set('port', PORT);
app.set('host', 'localhost');
app.set('views', __dirname + '/views');
app.set('view engine', 'ejs');
let bodyParser = require('body-parser');
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(bodyParser.json());
app.use(express.static(path.join(__dirname, 'public')));
let session = require('express-session');
app.use(session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: true,
cookie: { secure: true }
}));
app.get('/', function(req, res) {
res.render('index');
});
// use app.post to authenticate the signed request send from salesforce
app.post('/authenticate', function(req, res) {
let bodyArray = req.body.signed_request.split(".");
let consumerSecret = bodyArray[0];
let encoded_envelope = bodyArray[1];
let check = crypto.createHmac("sha256", config.CONSUMER_SECRET).update(encoded_envelope).digest("base64");
if (check === consumerSecret) {
envelope = JSON.parse(new Buffer.from(encoded_envelope, "base64").toString("ascii"));
req.session.salesforce = envelope;
res.render('index', {
title: envelope.context.user.userName,
signedRequestJson: JSON.stringify(envelope)
});
}
});
//Get the certificates
let sslkey = fs.readFileSync('ssl-key.pem');
let sslcert = fs.readFileSync('ssl-cert.pem');
let options = {
key: sslkey,
cert: sslcert,
requestCert: false,
rejectUnauthorized: false,
agent: false
};
https.createServer(options, app).listen(app.get('port'), function(){
console.log('info','Express server listening on port ' + app.get('port'));
});
Resources : Salesforce Canvas App Documentation