This article is aimed for beginners who want to create a simple node.js application. In this example will be using VS Code. Below are the steps –
1. Install node.js for your platform
2. Create an empty folder and open it in VS Code
3. Create a new file, app.js in your folder
4. Initialize npm and install express module. Open terminal and run below commands:
- npm init -y
- npm install express
Note: A package.json file will be created and node modules will be installed. Following will be the project structure:
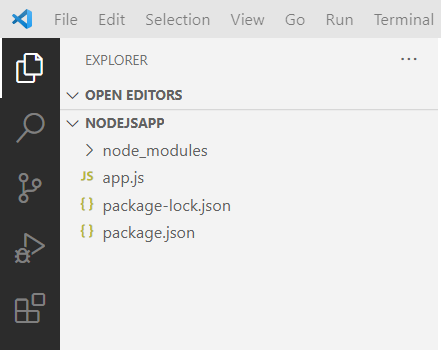
6. Open the app.js file and write the below code –
// Requiring module
const express = require('express');
// Creating express object
const app = express();
// Handling GET request
app.get('/', (req, res) => {
res.send('Node.js App is running on this server!')
res.end()
})
// Port Number
const PORT = process.env.PORT ||1884;
// Server Setup
app.listen(PORT,console.log(
`Server started on port ${PORT}`));
7. Run node app.js in the terminal. Server will start running at port 1884
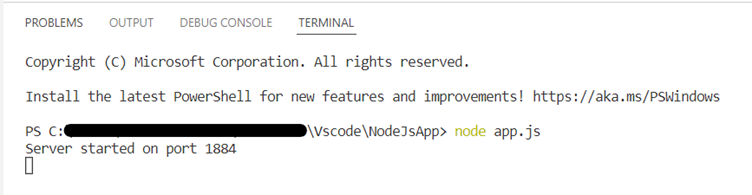
8. In web browser go to http://localhost:1884/, you will see the following output:
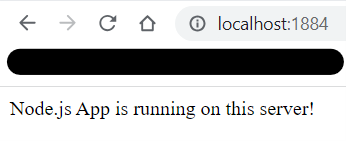
9. To render the UI file, first install a view engine. Here, we are using ejs.
- npm install ejs – for view engine as ejs
- Create a views folder
- Create a .ejs file under views folder
- Add html content inside the index.ejs file and save
- Use the render() method in your app.js
- app.get(‘/’, function(req, res) { res.render(‘index’); });
- Run node app.js in the terminal
- In web browser go to http://localhost:1884/, you will see the following output:
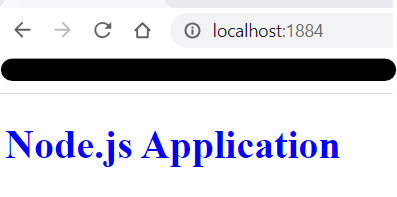
10. Below are the code snippet for app.js and index.ejs
// Requiring module
const express = require('express');
// Creating express object
const app = express();
// Port Number
const PORT = process.env.PORT ||1884;
// all environments
app.set('port', PORT);
app.set('host', 'localhost');
app.set('views', __dirname + '/views');
app.set('view engine', 'ejs');
app.get('/', function(req, res) {
res.render('index');
});
// Server Setup
app.listen(PORT,console.log(
`Server started on port ${PORT}`));
<!DOCTYPE html>
<html>
<body>
<h1 style="color:blue;">Node.js Application</h1>
</body>
</html>